Course curriculum
-
-
Welcome
-
Bootcamp Objective
-
Bootcamp Logistics
-
Audience Q&A
-
What does a Java backend engineer do?
-
The three tier architecture
-
The CDN and how it works
-
The REST API request flow
-
Audience Q&A on REST and HTTP
-
Cookies and sessions with HTTP
-
Essential tools of the trade
-
Audience Q&A on app servers
-
Source control
-
Introducing the GitHub workflow
-
Audience Q&A on GitHub
-
Homework
-
Audience QA on SSR
-
Understanding the GitHub workflow
-
-
-
Data Types
-
Wrapper classes
-
Operators
-
Hands-on exercises
-
Arrays
-
Classes
-
Encapsulation
-
Inheritance and polymorphism
-
The static keyword
-
Why is main static?
-
Static vs instance methods
-
Nested classes
-
Inner class types
-
Records
-
Homework
-
Audience Q&A
-
-
-
Collections overview
-
Equality and the equals method
-
Rules for equality
-
Walkthrough of an equals implementation
-
Hashing and hash codes
-
Equality and hash codes
-
Hands-on exercise
-
Time complexity
-
Space complexity and tradeoffs
-
Comparator and Comparable
-
The iterator pattern
-
Fail fast iterators
-
The Collection interface hierarchy
-
The List interface
-
Amortized time complexity
-
LinkedList
-
Vector
-
Autoboxing with Lists
-
CopyOnWriteArrayList
-
List iterators
-
SubList
-
Equals with List objects
-
The Set interface
-
HashSet
-
HashSet load factor and capacity
-
LinkedHashSet
-
SortedSet and TreeSet
-
TreeSet exercise
-
NavigableSet
-
The Map interface
-
The Queue interface
-
The Deque interface
-
WeakHashMap
-
Collections utility methods
-
-
-
1 Functional programming in Java
-
2 What is functional programming_
-
3 Functions vs methods
-
4 Limitations of Object Oriented Programming
-
5 First class functions
-
6 Introducing Lambda expressions
-
7 Lambda expression examples
-
8 Recommendation and Q&A
-
9 Functional interfaces
-
10 Typing lambdas with interfaces
-
11 Why Single Abstract Method_
-
12 Lambda hands-on coding
-
13 Interface typing, not SAM typing
-
14 Revisiting the task example with lambdas
-
15 The FunctionalInterface annotation
-
16 Lambda code demo
-
17 Lambdas vs anonymous classes
-
18 The interface overhead of lambdas
-
19 JDK functional interfaces
-
20 The Function interface
-
21 Consumer, Supplier and Predicate
-
22 JDK functional interfaces hands-on
-
23 Function arity
-
24 Operator interfaces
-
25 Method references
-
26 Method reference examples
-
27 Method references hands-on
-
28 Lambda calling lambdas calling lambdas
-
29 Composability with andThen and compose
-
30 Closures in Java
-
31 Pure functions
-
32 What are streams_
-
33 Declarative model
-
34 Stream API characteristics
-
35 The assembly line analogy
-
36 Streams code demo
-
37 Other ways to create streams
-
38 The toList method
-
39 Three key elements in Streams API
-
40 Streams vs collections
-
41 Limit and filter operators
-
42 The map operator
-
43 The peek operator and debugging
-
44 The effect of terminal operations
-
45 distinct and sorted operators
-
46 Concatenating streams
-
47 Working with object streams
-
-
-
A typical Java project needs
-
How Maven helps
-
Project management with Maven
-
Convention over configuration
-
The Project Object Model
-
Audience question about classpath
-
Creating a Maven project in IntelliJ
-
Examining pom.xml
-
Maven project coordinates
-
Running Maven targets
-
Dependency Management
-
Maven repositories
-
Fetching a dependency from Maven Central
-
Dependency Scopes
-
Maven Versioning
-
Maven Plugins and Goals
-
-
-
Building the time tracker app
-
Outlining the CLI experience
-
Designing the data structures
-
Coding start and stop tasks
-
Accepting CLI arguments
-
Setting up and running start task
-
Refactor argument processing
-
Switching to the new parser
-
Running and troubleshooting
-
Saving task information to file
-
Refactoring all file related code into FilesUtil
-
Add reporting for tasks
-
Report categories
-
Wrap up
-
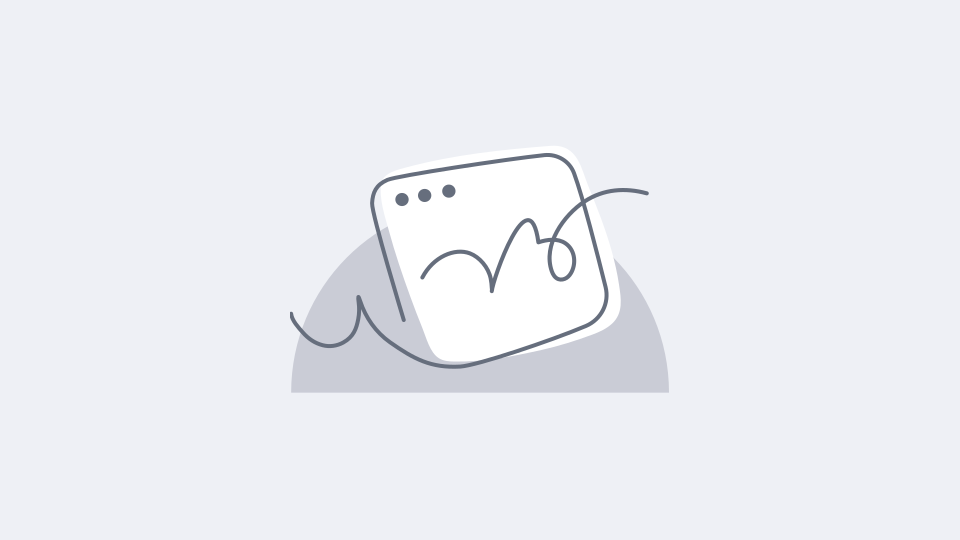
About this course
- Free
- 175 lessons
- 21 hours of video content